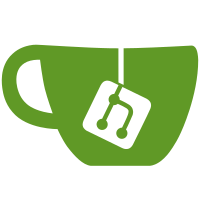
Adds support for several special characters in identifiers. Adds a demo for converting values to source code and another for checking equality. Updates the existing demo and tests to reflect new names for functions returning booleans.
47 lines
2.1 KiB
Plaintext
47 lines
2.1 KiB
Plaintext
-- Thanks to intensionality, we can inspect the structure of a given value
|
|
-- even if it's a function. This includes lambdas which are eliminated to
|
|
-- Tree Calculus (TC) terms during evaluation.
|
|
|
|
-- Triage takes four arguments: the first three represent behaviors for each
|
|
-- structural case in Tree Calculus (Leaf, Stem, and Fork).
|
|
-- The fourth argument is the value whose structure is inspected. By evaluating
|
|
-- the Tree Calculus term, `triage` enables branching logic based on the term's
|
|
-- shape, making it possible to perform structure-specific operations such as
|
|
-- reconstructing the terms' source code representation.
|
|
triage = (\a b c : t (t a b) c)
|
|
|
|
-- Base case of a single Leaf
|
|
sourceLeaf = t (head "t")
|
|
|
|
-- Stem case
|
|
sourceStem = (\convert : (\a rest :
|
|
t (head "(") -- Start with a left parenthesis "(".
|
|
(t (head "t") -- Add a "t"
|
|
(t (head " ") -- Add a space.
|
|
(convert a -- Recursively convert the argument.
|
|
(t (head ")") rest)))))) -- Close with ")" and append the rest.
|
|
|
|
-- Fork case
|
|
sourceFork = (\convert : (\a b rest :
|
|
t (head "(") -- Start with a left parenthesis "(".
|
|
(t (head "t") -- Add a "t"
|
|
(t (head " ") -- Add a space.
|
|
(convert a -- Recursively convert the first arg.
|
|
(t (head " ") -- Add another space.
|
|
(convert b -- Recursively convert the second arg.
|
|
(t (head ")") rest)))))))) -- Close with ")" and append the rest.
|
|
|
|
-- Wrapper around triage
|
|
toSource_ = y (\self arg :
|
|
triage
|
|
sourceLeaf -- Triage `a` case, Leaf
|
|
(sourceStem self) -- Triage `b` case, Stem
|
|
(sourceFork self) -- Triage `c` case, Fork
|
|
arg) -- The term to be inspected
|
|
|
|
-- toSource takes a single TC term and returns a String
|
|
toSource = (\v : toSource_ v "")
|
|
|
|
exampleOne = toSource true -- OUT: "(t t)"
|
|
exampleTwo = toSource not -- OUT: "(t (t (t t) (t t t)) (t t (t t t)))"
|